OOP Fundamental
Object-Oriented Programming (OOP) ภาษาไทย: การเขียนโปรแกรมเชิงวัตถุ เป็นรูปแบบการเขียนโปรแกรมชนิดหนึ่ง ที่นักพัฒนาให้การยอมรับ และเป็นที่นิยม (ในอดีต) สำหรับโปรแกรมที่เริ่มมีความซับซ้อน โดยมีลักษณะเบื้องต้น
- การมองโปรแกรมเสมือนวัตถุหนึ่งอย่าง เช่น นก รถยนต์
- การมองค่า หรือตัวแปรของโปรแกรม เป็นคุณสมบัติ เช่น นกมีน้ำหนัก 2 kg, รถยนต์ มีความเร็ว 60 km/hr
- การมองฟังก์ชันการทำงานของโปรแกรม เป็น พฤติกรรม เช่น นกบินได้, รถยนต์สามารถวิ่งไปข้างหน้าได้
OOP Backbone
- Encapsulation การห่อหุ้มข้อมูล
- Abstraction การรู้แค่ที่จำเป็น
- Inheritance การสืบทอดคุณสมบัติ
- Polymorphism การมีหลายรูปแบบ
OOP Vocabulary
- Method คือ ฟังก์ชันของโปรแกรมนั้นๆ เปรียบเทียบกับพฤติกรรมหรือการทำงานของวัตถุ
- Attribute คือ ค่าที่ระบุให้วัตถุนั้นๆ เปรียบได้กับ ตัวแปร หรือ ค่าคงที่ของโปรแกรม
- Class คือ การสร้างชนิดของข้อมูลใหม่ ตามที่เราต้องการ โดยอาศัยหลักการทำงานของ OOP เป็นพื้นฐาน สามารถระบุ method, attribute ได้ตามที่ programmer ต้องการ
- Object คือ ข้อมูลที่เกิดขึ้น หลังจากสร้าง class แล้ว เราสามารถสร้าง object ที่มี attribute ที่ต่างกันได้ โดยใช้พื้นฐานจาก class เดียวกัน
- Blueprint คือสิ่งเดียวกับ class หลังจากสร้าง class แล้ว จะเปรียบเสมือนมีแม่แบบ ในการสร้าง Object ใดๆ ก็ได้ โดยใช้ method, attribute ของ class นั้นๆ
OOP in Python
python รองรับ OOP เต็มรูปแบบ มี syntax รองรับการสร้าง class, กำหนด attribute, method และยังรองรับการสืบทอด class ด้วย
Class
คือ แม่แบบ (Blueprint) ของการสร้างข้อมูลชนิดที่เราต้องการ สามารถกำหนดค่าและฟังก์ชันการทำงานตามที่ผู้ใช้ต้องการได้
# create blueprint of bird object
class Bird:
weight = 15
Objects
หลังจากที่เรามี class (แม่แบบ) แล้ว เราสามารถสร้าง object ขึ้นมาจาก class นี้ได้
# create blueprint of bird object
class Bird:
weight = 15
color = "red"
liverbird = Bird() # create object of liverbird
penguin = Bird() # create object of penguin
print(liverbird.weight) # show liverbird's weight (15kg)
print(penguin.color) # show penguin's color (red)
Constructor
เป็นการกำหนด Attribute ให้กับ object ทำให้ object แต่ละตัวมีค่าที่แตกต่างกันได้ โดย python จะได้ syntax function __init__
และ self
ในการกำหนด attribute
class Bird:
def __init__(self, weight, color):
self.weight = weight
self.color = color
liverbird = Bird(2, "white") # create object of liverbird
penguin = Bird(10, "black") # create object of penguin
print(liverbird.weight) # 2
print(penguin.color) # black
Representation
การกำหนดค่าที่แสดงเมื่อเราแสดงผล object นั้นเปล่าๆ ถ้ากรณีไม่กำหนด str ค่าที่เรา แสดงผลจะเป็นชนิดของ class นั้น ตามด้วย pointer ของ class
class Bird:
def __init__(self, weight, color):
self.weight = weight
self.color = color
def __str__(self):
return f"{self.weight} kg and {self.color})"
liverbird = Bird(2, "white") # create object of liverbird
penguin = Bird(10, "black") # create object of penguin
print(liverbird) # 2 kg and white
print(penguin) # 10 kg and black
Methods
เป็นการกำหนดการทำงาน (พฤติกรรม) ของ class ที่เราสร้างขึ้นมา ใช้หลักการเดียวกับฟังก์ชัน
class Bird:
def __init__(self, weight, color, can_fly):
self.weight = weight
self.color = color
self.can_fly = can_fly
def fly(self):
return "flying" if self.can_fly == True else "cannot fly!"
liverbird = Bird(2, "white", True) # create object of liverbird
penguin = Bird(10, "black", False) # create object of penguin
print(liverbird.fly()) # flying
print(penguin.fly()) # cannot fly!
Modify & Remove
class Bird:
def __init__(self, weight, color, can_fly):
self.weight = weight
self.color = color
self.can_fly = can_fly
liverbird = Bird(2, "white", True) # create object of liverbird
penguin = Bird(10, "black", False) # create object of penguin
# update properties
penguin.weight = 15
print(penguin.weight)
# remove properties
del liverbird.color
print(liverbird.color)
# remove object
del penguin
print(penguin)
Inheritance
เป็นการสืบทอด class โดยเราจะมองว่าโปรแกรมเชิงวัตถุ สามารถมีคุณสมบัติคล้ายคลึงกัน แต่เปลี่ยนแปลงบางอย่างได้ โดยจะเรียก class หลักว่า parent และ class ที่สืบทอดว่า child
โดย syntax ที่ต้องระบุเพิ่มได้แก่
- class ที่เป็น child ต้องระบุ class parent ตอนประกาศ class
- ถ้าต้องการสืบทอด properties และ attribute จาก parent ต้องใช้
super().__init__(self, ...)
ตอนสร้าง contructor ของ child
class Animal:
def __init__(self, weight, color):
self.weight = weight
self.color = color
def sleep(self):
print("sleeping")
class Bird(Animal):
def __init__(self, weight, color, can_fly):
# syntax for inherit method/props from parent
super().__init__(weight, color)
self.can_fly = can_fly
def fly(self):
return "flying" if self.can_fly == True else "cannot fly!"
# create object of cat
cat = Animal(5, "orange")
print(cat.weight)
cat.sleep()
# print(cat.fly())
# create object of penguin
penguin = Bird(10, "black", True)
print(penguin.weight)
penguin.sleep()
print(penguin.fly())
Exercise
- สร้าง class ของรถยนต์ (car) โดยมี properties และ method ดังนี้
- properties: สี, ชนิดของรถ, ความเร็ว (km/hr)
- method:
- ขับ (drive): ให้แสดงผล driving
- method คำนวนเวลาที่ใช้ในการเดินทาง: ให้รับค่า parameter เป็นระยะทาง จากนั้น ให้นำความเร็วมาหาร และแสดงผลเป็นเวลาที่ใช้ในการเดินทาง
- โจทย์
- ให้แสดงผลสีของรถยนต์ Benz
- ให้แสดงผลระยะเวลาที่ใช้ขับรถ BMW ถ้ากำหนดความเร็ว = 200, สี = black, ชนิดของรถ = sedan
- สร้าง class ของ Person กำหนดให้มีเพศ, อายุ, ชื่อจริง, นามสกุล จากนั้นให้สร้าง child class ชื่อ Student โดยให้สืบทอดคุณสมบัติของ Person แต่มีค่าที่เพิ่มมา คือ เลขประจำตัวนักเรียน และ ชั้นเรียน
- แสดงผล ข้อมูลบัตรประจำตัวนักเรียนเมื่อ
Student("male", 15, "Pathompat", "Sungpankhao", "67001202961", "grade 6")
- ทดสอบแสดงผลชั้นเรียนของ
Person("female", 23, "Malinee", "Kunthong")
- แสดงผล ข้อมูลบัตรประจำตัวนักเรียนเมื่อ
Reference
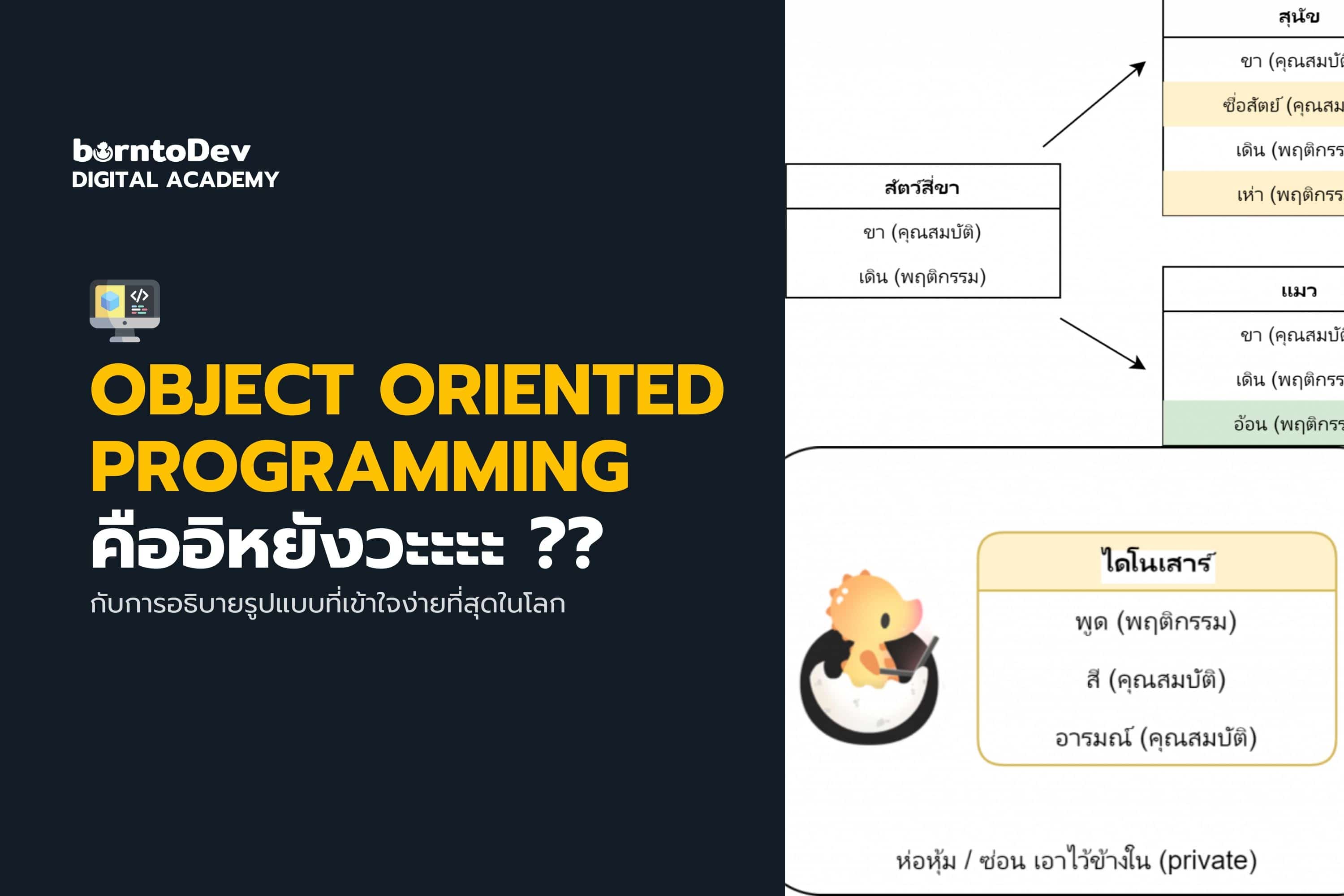

