Definition
ฟังก์ชันเป็นการรวมชุดของโค้ดสำหรับทำงานเฉพาะส่วนที่ต้องการ ผู้ใช้สามารถกำหนดชื่อของฟังก์ชัน ตัวแปรของฟังก์ชัน (parameters) และผลลัพธ์ของฟังก์ชัน (return value) ตามที่ออกแบบ และสามารถเรียกฟังก์ชันใช้งานซ้ำได้ตลอดหลังจากประกาศฟังก์ชันแล้ว
Syntax
# function
def function_name():
# code blocks in function
return value
# function with parameters
def function_name(param1, param2, ...):
# code blocks in function
return value
# calling function
function_name()
function_name(a, b)
Example
- ตัวอย่างฟังก์ชันสำหรับแสดงผลข้อมูล
def print_hello():
print("Hello")
print_hello() # print Hello
- ตัวอย่างฟังก์ชันสำหรับการบวกเลข
def sum(a, b):
return a + b
print(sum(5, 2)) # print 7
Parameters
- ไม่ต้องกำหนด type เหมือนตัวแปรปกติ
- สามารถใช้ร่วมกับ ตัวแปรปกติได้ แต่ชื่อตัวแปรห้ามซ้ำกับ parameter
- ถ้าประกาศ parameter จำนวนเท่าไหร่ ตอนเรียกใช้ฟังก์ชันต้องกำหนด parameter จำนวนให้เท่ากันเสมอ
def multiply(a, b):
c = a * b # can use variable with parameter
return c
multiply(1) # error
multiply(2, 3) # return 6
Arbitrary parameter
- ในกรณีท่ีเราไม่ทราบจำนวน parameter สามารถระบุเป็น *args, **kwarg ได้โดยที่
- *args จะเป็นการส่ง parameter โดยใช้ tuple
def my_func(*numbers):
print("3rd is " + numbers[2])
my_func(1, 2, 3, 4) # print 3rd is 3
- **kwargs จะเป็นการส่ง parameter โดยใช้ dictionary
def my_func(**user):
name = user["name"]
age = user["age"]
return name + " " + age
my_func(name = "Mung", age = 15) # print Mung 15
Default Parameter
- สามารถกำหนดค่าตั้งต้นของ parameter ได้ กรณีผู้ใช้ไม่ระบุค่ามา จะใช้ค่า default แทน
def my_func(a, b = 2):
c = a + b
return c
my_func(5, 3) # print 8
my_func(5) # print 7
Recursive function
- เป็นฟังก์ชันที่มีการเรียกใช้ฟังก์ชันซ้อนเข้าไปข้างในเรื่อยๆ เกิดเป็นการทำงานคล้ายการวนลูป
- ตัวอย่างการใช้งาน เช่น Fibonacci Number, Euclid’s Algorithm
def fibonacci(n):
if n < 0: # error
print("error")
elif n == 0: # Check if n is 0, then it will return 0
return 0
elif n == 1 or n == 2: # Check if n is 1,2, will return 1
return 1
else:
return fibonacci(n-1) + fibonacci(n-2)
print(fibonacci(9))
Lampda
- เป็น expression สำหรับเขียน function แบบรวดเร็ว ภายในบรรทัดเดียว
- สามารถใช้ร่วมกับฟังก์ชันปกติได้
- สามารถกำหนด parameters ได้ และต้อง return กลับไปในบรรทัดเดียว
Syntax
increment_func = lambda a : a + 10
print(increment_func(5))
Exercise
- สร้างฟังก์ชันสำหรับการบวก, คูณ, หาร เลข 2 ตัว โดยแสดงคำตอบ ดังนี้
- ผลบวกของ 44, 55 และ 71, 3
- ผลคูณของ 2, 5 และ 3, 10
- ผลหารของ 84, 2
- สร้างฟังก์ชันสำหรับคำนวณพื้นที่ของรูปเรขาคณิตดังนี้
- วงกลม
- รับค่า: รัศมี
- แสดงผล: โดยใช้สูตร
area = π×r**2
- สี่เหลี่ยม
- รับค่า: ความกว้าง, ความยาว
- แสดงผล: ใช้สูตร
area = width x height
- วงกลม
- Anagram Checker: ให้สร้างฟังก์ชันสำหรับเช็ค Text Input 2 ตัว ถ้าประกอบด้วยตัวอักษรที่เหมือนกัน (ไม่สนใจการเรียง) ให้แสดงผล
True
ถ้าไม่ใช่เงื่อนไขดังกล่าวให้แสดงผลFalse
anagrams("listen", "silent")) # True
anagrams("hello", "world")) # False
anagrams("hello", "hello")) # True
Reference
Python Program to Print the Fibonacci sequence - GeeksforGeeks
A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
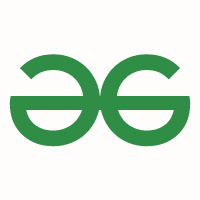
Euclidean algorithms (Basic and Extended) - GeeksforGeeks
A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
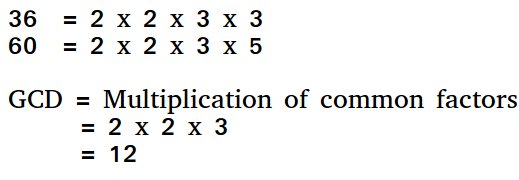
Python Program to Check if Two Strings are Anagram - GeeksforGeeks
A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
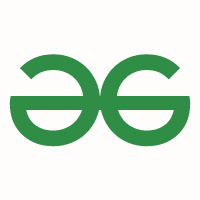