Basic
ในการเขียนโปรแกรม จะต้องมีการ "วนซ้ำ" เพื่อตรวจสอบข้อมูลจำนวนมากๆ หรือเพื่อใช้สั่งให้ทำงานหลายรอบ
Flowchart
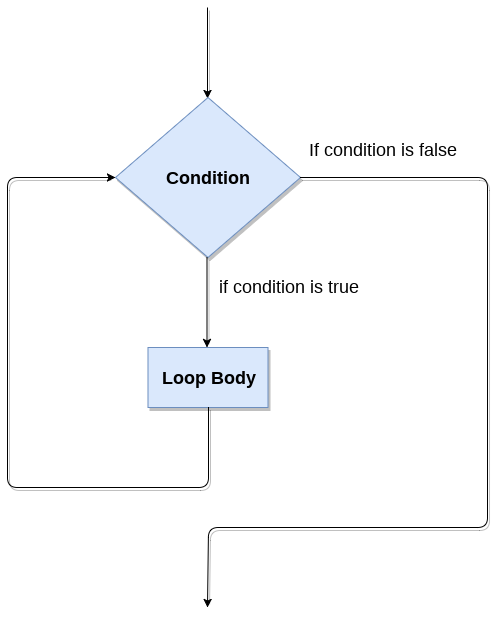
While Loop
Definition
เป็น loop พื้นฐานที่มีในทุกภาษา syntax ตรงไปตรงมา ทำงานจนไม่เข้าเงื่อนไขของ while จึงจะหยุดทำงาน
Syntax
i = 1 # define variable
while i < 6: # loop until i more than 5
print(i)
i += 1 # every loop increase i
Flowchart
Example
- รับ input ชื่อของผู้ใช้งานจนกว่าจะถึง 3 ชื่อจึงหยุดทำงานและแสดงผล
name = [] # store listname from input
while len(name) < 3: # need 3 names
inputName = input()
name.append(inputName)
# output
print(name)
print("list name has ", len(name), " names")
For Loop
Definition
เป็น loop ที่มีการประยุกต์ใช้หลากหลายมากกว่า while loop ส่วนมากจะนำมาใช้กับ Range, List, Tuple, Set เพราะสามารถวนลูปข้อมูลใน Collections ได้ง่าย และ Syntax ไม่ซับซ้อน
Syntax
# loop for range
for x in range(1, 5):
print(x)
# loop for list, set, tuple
listNum = [1, 2, 3, 4]
for x in listNum:
print(x)
Flowchart
Example
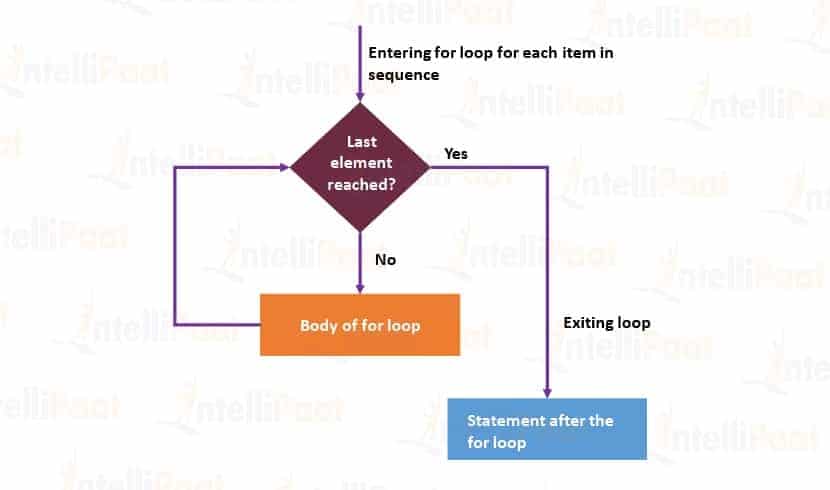
Break
ใช้หยุดการทำงานของ loop ก่อนจะถึงเงื่อนไขหลักของ for, while เช่น
names = ["Mung", "Peach", "Jones"]
# break for
for name in names:
print(name)
if name == "Peach":
break
# break while
i = 0
while i < len(names):
print(names[i])
if names[i] == "Peach":
break
i+=1
Pass
ในกรณีใน loop ไม่มีคำสั่งอะไรเลย ให้ใส่ pass ไว้เสมอ เช่น
names = ["Mung", "Peach", "Jones"]
# pass for
for name in names:
pass
Continue
กรณีให้ข้ามการทำงานไป loop รอบถัดไป เช่น
names = ["Mung", "Peach", "Jones"]
# skip display peach
for name in names:
if name == "Peach":
continue
print(name)
Nested Loop
- ใช้กับข้อมูลที่ซับซ้อนขึ้น เช่น List 2 มิติ, List ที่มี Dictionary อยู่ข้างใน
listRoom = [
[101, 102, 103, 104],
[201, 202, 203],
[300, 301, 302]
]
for floor, rooms in enumerate(listRoom):
for room in rooms:
print("floor = ", floor + 1, ", room = ", room)
Collections Loop
- การวนลูปข้อมูลของ List
# list of name
listName = ["Mung", "Too", "Khun", "Chai"]
for name in listName:
print(name)
- การวนลูปข้อมูลของ Dictionary แสดงทั้ง Key และ Value
# dictionary of personal information
person = {
"name": "Mung",
"age": 25,
"gender": "male",
"address": "Bangkok"
}
# loop for showing info
for key, val in person.items():
print(key, " = ", val)
- การวนลูปข้อมูล List ของ Dictionary (** เจอบ่อย)
# define a list of dictionaries
books = [
{
"title": "To Kill a Mockingbird",
"author": "Harper Lee",
"year": 1960
},
{
"title": "1984",
"author": "George Orwell",
"year": 1949
},
{
"title": "The Great Gatsby",
"author": "F. Scott Fitzgerald",
"year": 1925
}
]
# loop list dict
for book in books:
print("Title: ", book['title'])
print("Author: ", book['author'])
print("Year: ", book['year'])
print("-" * 20)
Exercise
- วาด flowchart และเขียนโปรแกรมรับค่าตัวเลข 5 ตัว หลังจากรับตัวเลขมาคำนวณเพื่อแสดงผล
- แสดงผลการบวกของตัวเลขทุกตัว
- ผลการคูณของตัวเลขทุกตัว
- เงื่อนไขเพิ่มเติม
- ถ้าตัวเลข input: เกิน 100 ให้แสดงผล
error: input number exceed limit
- ถ้าผลคูณหรือผลบวกเกิน 9999 ให้แสดงผล
error: output exceed limit
- ถ้าตัวเลข input: เกิน 100 ให้แสดงผล
- เขียนโปรแกรมรับค่าตัวเลข 1-12 จากนั้นแสดงผล ตารางสูตรคูณของตัวเลขนั้น ตัวอย่าง output ของ input 3
- 3 x 1 = 3
- 3 x 2 = 6
- ....
- 3 x 12 = 36
- เขียนโปรแกรมรับชุดตัวเลข ให้คำนวณว่าตัวเลขตัวใดเป็นเลขคู่หรือเลขคี่ จากนั้น แสดงผล
- ถ้าเป็นเลขคู่แสดงผล
Even numbers: n1, n2, n3
- ถ้าเป็นเลขคี่แสดงผล
Odds number: n4, n5, n6
- ถ้าเป็นเลขคู่แสดงผล
💡
Hint: ใช้ numbers = list(map(int, input().split(","))) เพื่อเก็บชุดของตัวเลข
Mini projects
เขียนโปรแกรมรับข้อมูล ชื่อเล่น, อายุ, ราศี, เลขมือถือตำแหน่งสุดท้าย ของผู้ใช้ โดยให้ประเมินค่าที่รับเข้ามาเป็นดวงในช่วงนี้ จากเกณฑ์ดังนี้
- กรุ๊ปเลือด
- ถ้ากรุ๊ปเลือด A, AB ให้คะแนน 5 คะแนน
- ถ้ากรุ๊ปเลือด B ให้คะแนน 3 คะแนน
- ถ้ากรุ๊ปเลือด O ให้คะแนน 1 คะแนน
- ถ้ากรุ๊ปเลือดไม่ได้อยู่ในรายการนี้ให้แสดงผล
error blood type
- อายุ
- ถ้าอายุหาร 7 ลงตัว ให้คะแนน 5 คะแนน
- ถ้าอายุหาร 15 ลงตัว ให้คะแนน 3 คะแนน
- ถ้าหาร 2 เคสข้างบนไม่ลงตัว ให้คะแนน 1 คะแนน
- ราศี: กำหนดคะแนนของราศีตาม dictionary นี้ ให้บวกคะแนนตามรายการข้างล่าง ถ้า input ไม่ได้อยู่ในรายการนี้ให้แสดงผล
error input zodiac
zodiac = {
'Aries': 4,
'Taurus': 2,
'Gemini': 5,
'Cancer': 1,
'Leo': 3,
'Virgo': 0,
'Libra': 4,
'Scorpio': 2,
'Sagittarius': 5,
'Capricorn': 1,
'Aquarius': 0,
'Pisces': 3
}
- เลขมือถือตำแหน่งสุดท้าย ให้ map ตัวเลขกับ List คะแนนนี้
pointForLastNum = [1, 2, 5, 0, 3, 4, 2, 3, 4]
- การแสดงผล ตามผลรวมของตัวเลข
- 15-20 คะแนน ให้แสดงผล
you might get a good luck soon.
- 8-14 คะแนน ให้แสดงผล
you have normal luck.
- คะแนนต่ำกว่า 7 ให้แสดงผล
ahh, i hope that not worst.
- 15-20 คะแนน ให้แสดงผล
- การเก็บข้อมูล
- ให้บันทึกค่าทั้งหมดของ user รวมถึงคะแนนที่ได้ใส่เข้า list, dictionary ไว้
- ถ้ากรณี user input ข้อมูลชื่อที่มีอยู่ในระบบแล้ว ให้แสดงผลข้อมูลคะแนนของรอบล่าสุดไว้
Reference
W3Schools.com
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.

W3Schools.com
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.

How to access the index value in a ‘for’ loop?
How do I access the index while iterating over a sequence with a for loop?
xs = [8, 23, 45] for x in xs: print("item #{} = {}".format(index, x)) Desired output:
item #1 = 8
item #2…
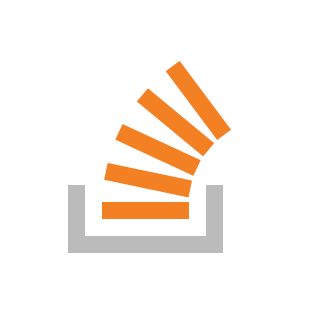