Conditional Programming
ในการเขียนโปรแกรมมักมีเงื่อนไขในการทำงาน เพื่อให้โปรแกรมทำงานหรือแสดงผลลัพธ์ต่างกันตามเงื่อนไขที่กำหนด
Flowchart
แผนภาพที่ระบุลำดับการทำงาน และเงื่อนไขการทำงานของโปรแกรม
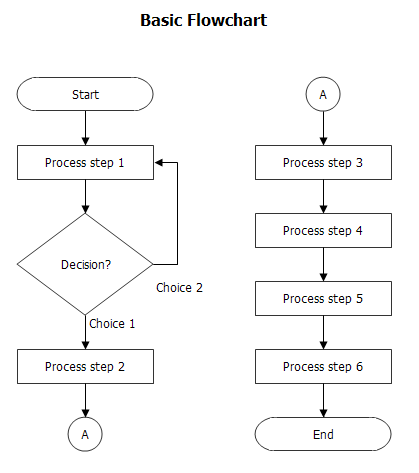
- วงกลม: กำหนดจุดเริ่มต้น - สิ้นสุดของโปรแกรม
- สี่เหลียมผืนผ้า: การทำงานของโปรแกรม
- สี่เหลี่ยมข้ามหลามตัด: เงื่อนไขของโปรแกรม
Conditional Syntax
💡
ตอนที่เริ่มเขียน condition จะมีเรื่องเกี่ยวกับ indent และ closing bracket ที่ต้องดูเพิ่มเติมด้วย
- If Statement
a = 10 # assign variable
if a > 5:
print("a is more than 5.")
example code [1]
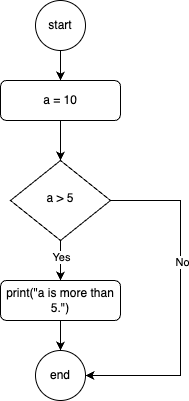
- If-Else statement
a = 2 # assign variable
if a > 5:
print("a is more than 5")
else:
print("a is less than or equal 5")
example code [2]
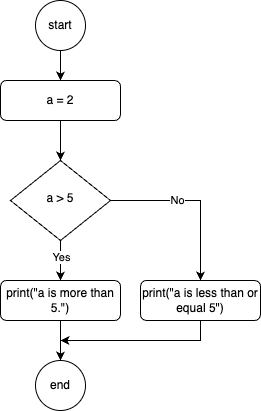
- If-Else If statement
a = 12 # assign variable
if a < 5:
print("a is more than 5")
elif a >= 5 and a <= 15:
print("a is between 5 and 15")
else:
print("a is less than or equal 5")
example [3]
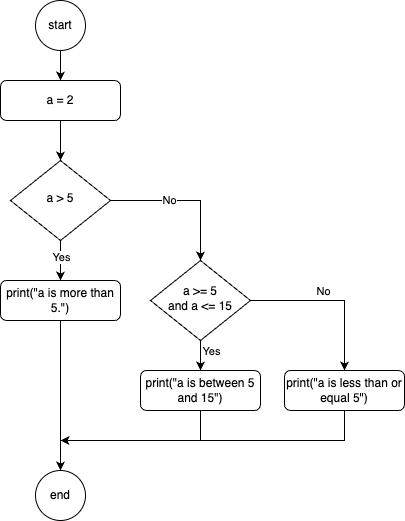
Nested Condition
if, else, else-if สามารถเขียนเงื่อนไขซ้อนกันได้ ขึ้นอยู่กับการทำงานของโปรแกรม
a = 12 # assign variable
if a > 5: # a is more than 5
if a < 8:
print("a is between 5 and 7")
elif a >= 8 and a <= 12:
print("a is between 8 and 12")
else:
print("a is greather than 12")
else: # a is less than 5
print("a is less than 5")
example code nested if
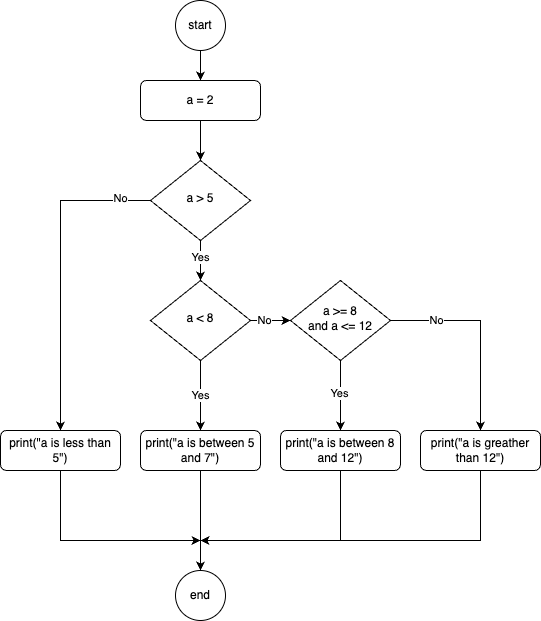
Additional Syntax
- Switch case statement
- ใช้สำหรับเคสที่เงื่อนไขไม่ซับซ้อนแต่มีหลายๆ เงื่อนไข
- สามารถเขียนแทนด้วย if ปกติได้
- ใช้ได้เฉพาะ Python version 3.12 ขึ้นไป
http_code = "418"
match http_code:
case "200":
print("OK")
case "404":
print("Not Found")
case "418":
print("I'm a teapot")
case _:
print("Code not found")
- Shorthand if statement
- ใช้สำหรับเงื่อนไขง่ายๆ ไม่ซับซ้อน
- ใช้สำหรับผลลัพธ์การทำงานที่มีคำสั่งเดียว
a = 2
b = 330
print("A") if a > b else print("B")
Excercise
- จากโปรแกรมรับชื่อ นามสกุล อายุ ของบทเรียนที่แล้ว ให้เพิ่มเงื่อนไขการแสดงผลต่อไปนี้
- ถ้าหากอายุที่รับมาเกิน 18 ปีเกินแสดงผล
you can access this program
- ถ้าอายุไม่ถึง 18 ปีให้แสดงผล
sorry, you can't access this program
- ถ้าหากอายุที่รับมาเกิน 18 ปีเกินแสดงผล
- เขียน flowchart และโปรแกรมคำนวณเกรด โดยอิงจาก input ข้อมูลของ user โดยรับผลคะแนนเข้ามาเป็นตัวแปรและแสดงผลเป็นเกรดโดยที่
- หากคะแนนต่ำกว่า 50 คะแนน แสดงผลเกรด
F
- หากคะแนนอยู่ระหว่าง 51-60 แสดงผลเกรด
D
- หากคะแนนอยู่ระหว่าง 61-70 แสดงผลเกรด
C
- หากคะแนนอยู่ระหว่าง 71-80 แสดงผลเกรด
B
- หากคะแนนสูงกว่า 80 แสดงผลเกรด
A
- หากคะแนนต่ำกว่า 50 คะแนน แสดงผลเกรด
- เขียนโปรแกรมจาก flowchart นี้
Reference
W3Schools.com
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.

Python คำสั่ง แบบ Switch-Case Statements จะใช้ร่วมกับกัน ได้เเล้ว
คำสั่งแบบ Switch-Case Statements จะใช้สามารถใช้งานร่วมกับ Python กัน ได้เเล้ว พบกับเนื้อหาข้างใน สดใหม่+เเน่นๆ คลิกเลย
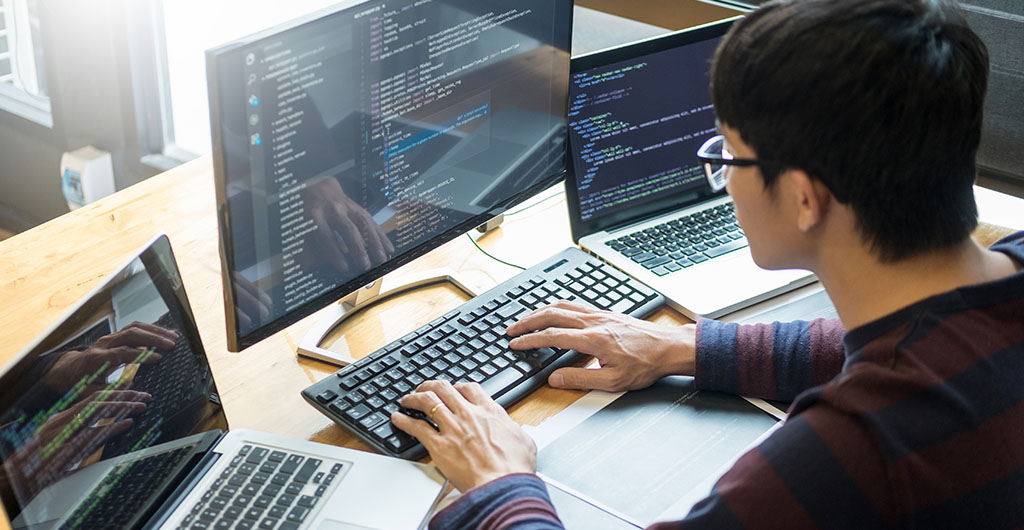