Sequencial search
- เรียกอีกอย่างว่า linear search
- เป็นการไล่หาข้อมูลทีละตัว โดยวนลูป
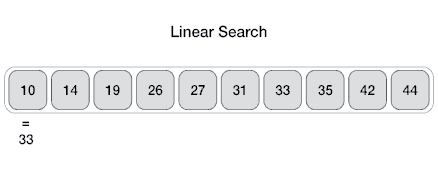
Example code
#include <stdio.h>
int main() {
int list[] = { 5, 1, 6, 12, 4, 3, 8, 33, 16, 21 };
int key = 18;
int size = sizeof(list) / sizeof(list[0]);
int count = 0;
for(int i = 0; i < size; i++) {
if (list[i] == key) { // compares each element of the array
printf("found at index %d\n", i + 1);
count = count + 1;
}
}
if (count == 0) {
printf("not found\n");
}
}
Binary search
- เป็นการหาข้อมูลทีละครึ่ง
- ข้อมูลที่ตั้งต้นต้อง Sorting ก่อน
- Time complexity (Big-O) =
log2n
Instruction
- ให้นำข้อมูลที่ Sorting มาเป็นค่าตั้งต้น โดยกำหนด
- target = ค่าที่ต้องการ search
- low = index array ตำแหน่งแรก
- high = index array ตำแหน่งสุดท้าย
- mid = index array ตำแหน่งตรงกลาง
(low + high) / 2
- เปรียบเทียบค่า target กับค่า mid ไปเรื่อยๆ
- ถ้า
target < ค่าของ mid
=> ปรับhigh = mid - 1
- ถ้า
target > ค่าของ mid
=> ปรับlow = mid + 1
- ถ้า
- ทำแบบนี้ไปเรื่อยๆ จน
low > high
จึงหยุดทำงาน
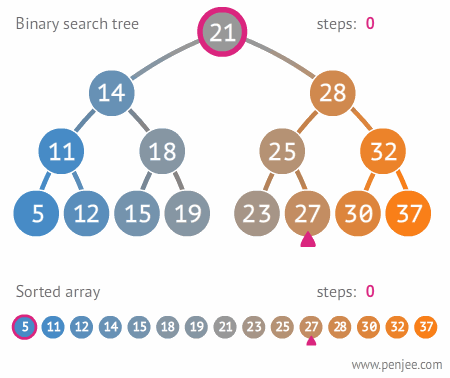
Example code
#include <stdio.h>
int main() {
int list[] = { 1, 5, 7, 8, 11, 13, 18, 21, 33, 35, 41, 52 };
int target = 35;
int size = sizeof(list) / sizeof(list[0]);
int first, mid, last, res;
first = 0;
last = size - 1;
res = -1;
while (first <= last) {
mid = (first + last) / 2;
if (list[mid] == target) {
res = mid;
break;
} else if (target > list[mid]) {
first = mid + 1;
} else if (target < list[mid]) {
last = mid - 1;
} else {
first = last + 1; // force end
}
}
printf("index = %d", res);
}
Sequencial search VS binary search
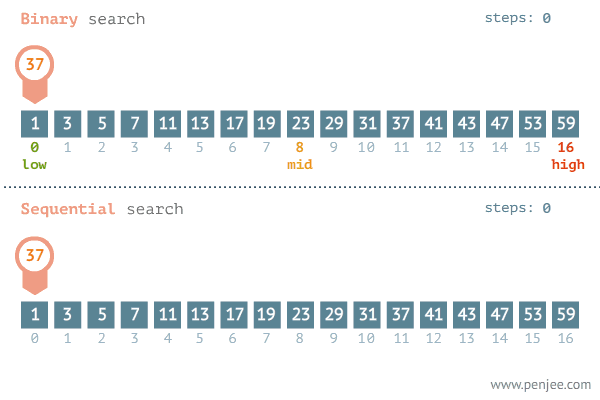
Reference
Sequential Search vs Binary Search
มีข้อมูลตั้งเยอะแยะ อยากหาข้อมูลที่ต้องการ ให้ได้เร็วๆ ทำยังไงดี มาดูกัน!!!

W3Schools.com
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
