Iteration
💡
การวนซ้ำ (Iteration) หรือ วนลูป (Loop) เป็นพื้นฐานของการเขียนโปรแกรม ที่ทำให้ process เกิดการทำงานซ้ำจนกว่าจะมีเงื่อนไขใดๆ ทำให้ผ่านการทำงานจุดนั้นไปได้ เป็นการใช้ประโยชน์จากความเร็วในการคำนวณของคอมพิวเตอร์ได้ และสามารถทำงานแทนคนในกรณีต้องคิดหลายๆ รอบได้เช่นกัน (เช่น 100 หรือ 10,000 รอบ)
Iteration flowchart
- ทำไมต้องใช้ flowchart?: อธิบายลำดับการทำงานที่มีการย้อนกลับไปทำซ้ำที่จุดเดิมได้
- Flowchart ที่ดีช่วยให้คนเขียนโปรแกรมออกแบบการทำงานได้ก่อนจะเขียนโค้ดจริง ลดการผิดพลาดหรือสับสนตอนที่เขียนโค้ด
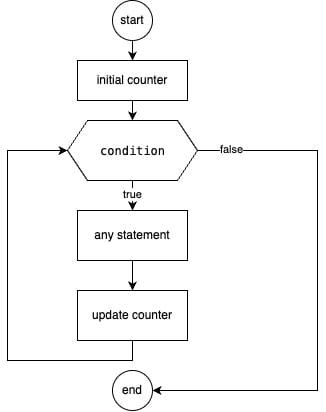
- flowchart ของ loop/iteration จะมีลักษณะทั่วไปคือ
- มีการ initial ค่าตั้งต้น (อาจจะไม่มีก็ได้) เพื่อนับหรือใช้อัพเดทค่าใน loop
- กำหนด condition ในการทำงาน ถ้าเข้าเงื่อนไข condition จะทำงานซ้ำไปเรื่อยๆ
- update ค่าภายใน (อาจจะไม่มีก็ได้) เพื่ออัพเดทค่าในทุกๆครั้งที่ loop ทำงาน และไว้ป้องกัน infinity loop ด้วย
Loop in C/C++
การวนซ้ำในภาษา c/c++ จะมี syntax ให้ใช้งานหลักๆ 3 ชนิด คือ
- do...while (Post-test)
- while (Pre-test)
- for (Pre-test)
ซึ่งแต่ละชนิดเหมาะกับการทำงานต่างกันตามที่ผู้ใช้ออกแบบโปรแกรม แต่หลักๆจะใช้ for เพราะง่ายต่อการจัดการชุดข้อมูล (Array)
Do..While Loop
- จะเริ่มทำงาน statement ก่อน แล้วจึงมาเช็คเงื่อนไขการวนซ้ำ
- เหมาะกับงานที่ต้องการให้ทำบางอย่างก่อนเข้า loop หรือ เริ่ม loop รอบต่อไป
- flowchart การทำงานจะต่างกับ for, while
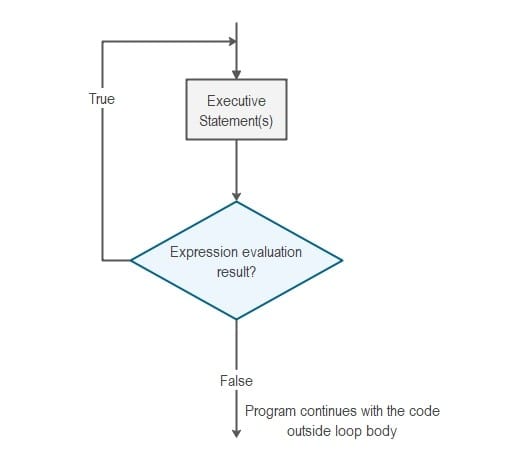
Syntax
do {
// statement
} while (condition);
Example
- แสดงผลตัวเลข 1-5
Code
#include <stdio.h>
int main() {
int i = 1;
do {
printf("%d\n", i);
i++;
} while (i <= 5);
return 0;
}
Flowchart
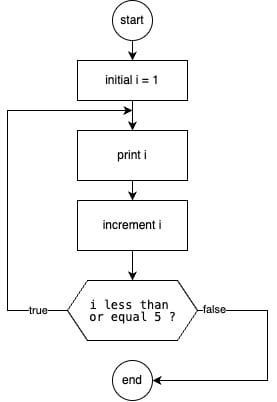
- รับ input ตัวเลขมาบวกกัน จนกว่า input จะเป็น EOF จึงจะแสดงผลบวกของตัวเลขทั้งหมด
Code
#include <stdio.h>
int main() {
int x = 0;
int sum = 0;
int testEOF;
do {
testEOF = scanf("%d", &x);
if (testEOF != EOF){
sum += x;
}
} while (testEOF != EOF);
printf("\nTotal: %d\n", sum);
return 0;
}
Flowchart
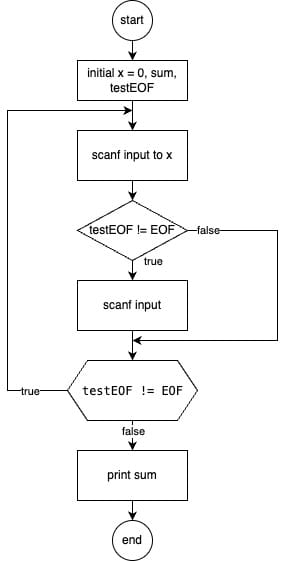
While loop
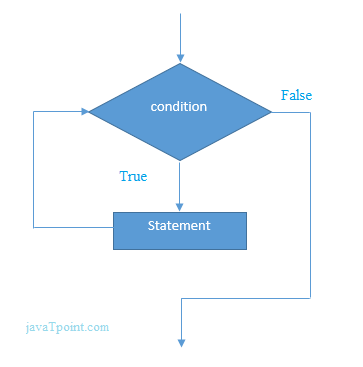
- เป็น loop pre-test (เช็คเงื่อนไขก่อนทำงาน)
- ส่วนมากใช้สำหรับเช็คเงื่อนไขที่มีตัวแปรภายนอกมาเกี่ยวข้อง เช่น การนับเวลา, การนับ input ที่กรอก
- ทำงานคคล้าย do...while เปลี่ยนแค่ลำดับการเช็คเงื่อนไขของลูปมาไว้ตอนแรก
Syntax
while() {
// statement
}
Example
- แสดงผลตัวเลข 1-5
#include <stdio.h>
int main() {
int i = 1;
while (i <= 5) {
printf("%d\n", i);
i++;
}
return 0;
}
Flowchart
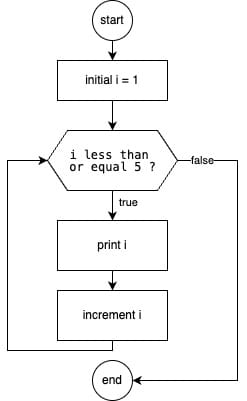
- แสดงผลตัวเลข n ไปจนถึง 1 เมื่อรับค่าตัวเลข 1-100 เท่านั้น
#include <stdio.h>
int main() {
int lineCount = 0;
int num;
printf("Please enter num 1 - 100: ");
scanf("%d", &num);
if (num > 100){
num = 100;
}
while (num > 0) {
// enter new line every 10 num
if (lineCount < 10){
lineCount++;
} else {
printf("\n");
lineCount = 1;
}
// print num
printf("%4d", num--);
}
return 0;
}
Flowchart
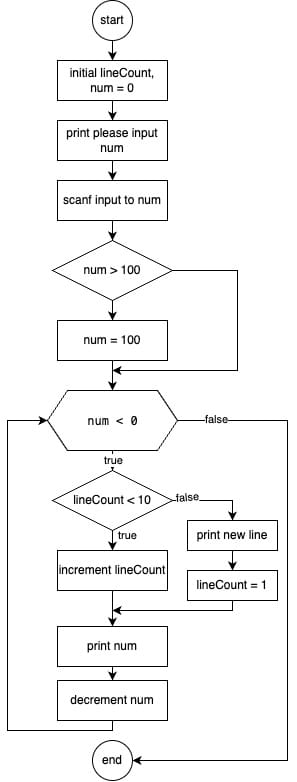
For loop
- เป็น pre-test loop
- flowchart การทำงานเหมือน while
- ย่อบาง statement ที่ต้องใช้กับการวนซ้ำ มาอยู่ข้างใน for เลย
- step การประกาศค่าตัวแปรตั้งต้น (initial counter)
- step การ update ค่าตัวแปร ตอนลูปจบ 1 รอบ
- เหมาะกับการใช้งานลูปที่มีขนาดของข้อมูลชัดเจน หรือ กำหนดรอบการวนซ้ำไว้ตั้งแต่แรก
Syntax
for (initialize; expression; update) {
// statement
}
// example
for (int i = 0; i < 5; i++) {
printf("%d\n", i);
}
- initialize: ทำตอนก่อนลูปเริ่ม ส่วนมากจะใช้กำหนดตัวแปร counter เช่น int i = 0, int j = 1;
- expression: เป็นเงื่อนไขในการทำงานของลูปครั้งถัดไป เช่น i < 5, i <= a.length()
- update: เป็นการทำงานตอนจบลูปแต่ละรอบ ส่วนมากใช้อัพเดทค่าตัวแปร counter เช่น i++, j += 2
Example
- แสดงผลตัวเลข 1-5
#include <stdio.h>
int main() {
for (int i = 1; i <= 5; i++) {
printf("%d\n", i);
}
return 0;
}
Flowchart เดียวกับ while
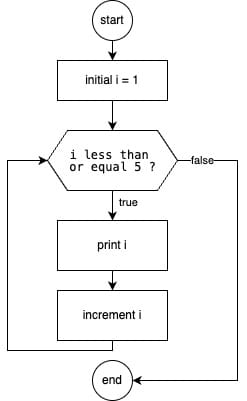
Nested Loop
- ลูปสามารถมีมากกว่า 1 ชั้นได้
- ต้องคำนึงถึงด้าน performance ด้วย ถ้ากรณี loop เกิน 2 ชั้น (Big-O)
#include <stdio.h>
int main() {
for (int i = 1; i <= 5; i++) {
printf("%d: ", i);
for (int j = 1; j < 10; j++){
printf("%d ", j);
}
printf("\n");
}
return 0;
}
Flowchart
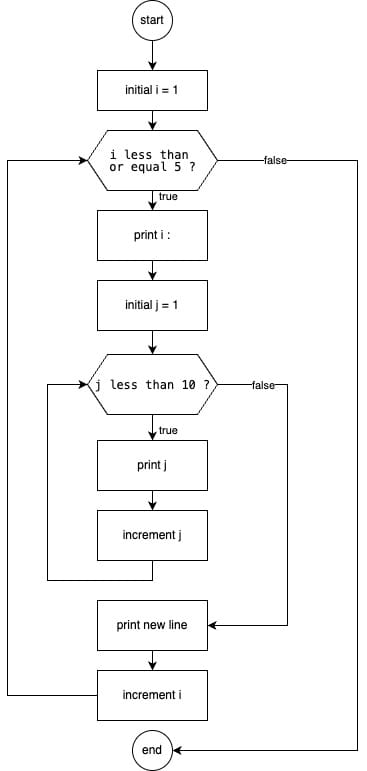
Exercise
- เขียนโปรแกรมรับค่าตัวเลข 1-12 จากนั้นแสดงผล ตารางสูตรคูณของตัวเลขนั้น ตัวอย่าง output ของ input 3
- 3 x 1 = 3
- 3 x 2 = 6
- ....
- 3 x 12 = 36
- เขียนโปรแกรมรับชุดตัวเลขกี่ตัวก็ได้ (จนกว่าจะเจอะ EOF) ให้คำนวณว่าตัวเลขตัวใดเป็นเลขคู่หรือเลขคี่ จากนั้น แสดงผล
- ถ้าเป็นเลขคู่แสดงผล
Even numbers: n1, n2, n3
- ถ้าเป็นเลขคี่แสดงผล
Odds number: n4, n5, n6
- ถ้าเป็นเลขคู่แสดงผล
Reference
W3Schools.com
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.

What Are the Types, Uses, and Benefits of Loops in Flowcharts?
You must know even the slightest details of looping in flowcharts if you want to employ them in programming flowcharts for repetitive operations.
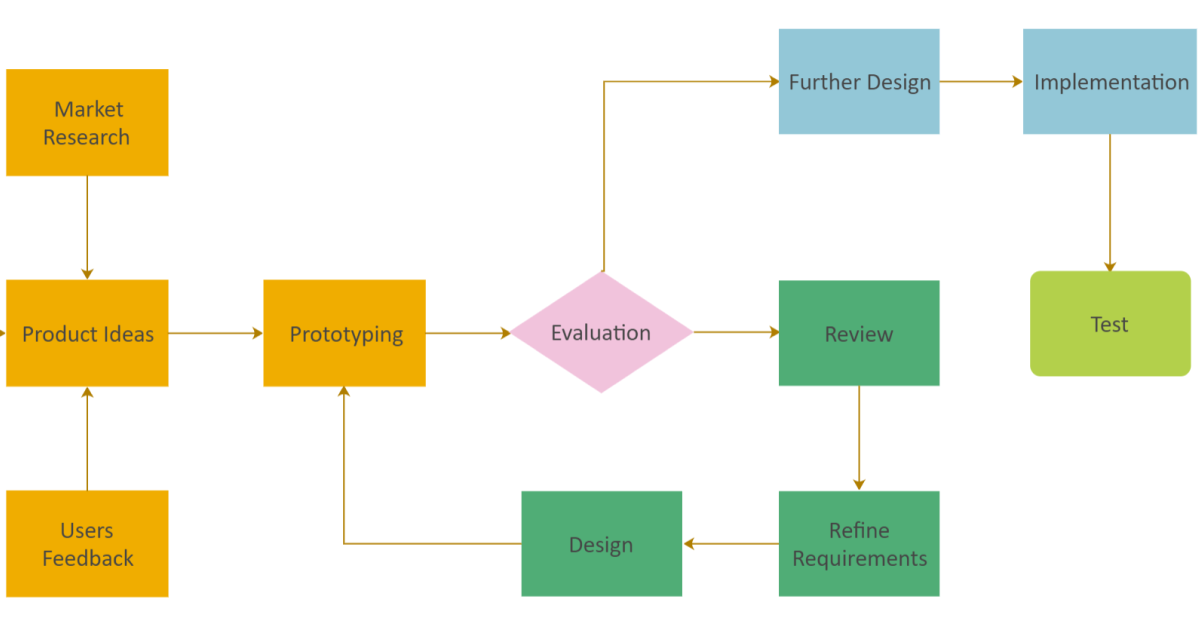
Iteration in C - javatpoint
Iteration in C with Tutorial, C language with programming examples for beginners and professionals covering concepts, c pointers, c structures, c union, c strings etc.
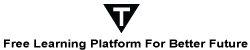